OpenCourse: Programming Paradigms / Stanford
「Programming Paradigms」コースは、スタンフォード大学が公開している講座です。「Programming Methodology」コースと「Programming Abstractions」コースのさらに上級に位置するコースです。
CおよびC++における、より高度なメモリ管理、Lispを用いた関数型パラダイムや、C/C++を用いたコンカレントプログラミング、そしてPython、Objective-C、C#などの言語についても学びます。
コースについての詳細やハンドアウトなどの関連資料は、「Stanford School of Engineering - Stanford Engineering Everywhere」から参照できます。
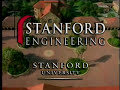
Languages and Paradigms Taught - C++ vs. Pure C, Procedural Paradigm vs. Object-Oriented Paradigm
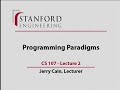
C/C++ Data Types - Interpretations, Sizes, Bits- How Bytes are Broken Up into Bits
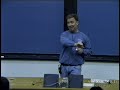
Converting Between Types of Different Sizes and Bit Representations Using Pointers, Little Endian vs. Big Endian
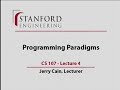
Creating a Generic Swap Function for Data Types of Arbitrary Size
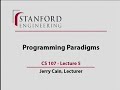
Generic Lsearch - Prototype, Comparison Function, Implementation
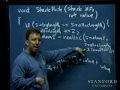
Integer Stack Implementation - Constructor and Destructor, Stackpush Implementation
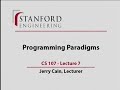
Problems with Ownership of Memory, How Default Implementation of Stackdispose Does Not Free Dynamically Allocated Data
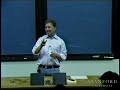
Heap Management - How Information about Allocations are Stored in the Heap
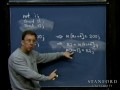
How a Code Snippet is Translated into Assembly Instructions, Store, Load, and ALU Operations
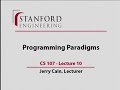
More Detail about Activation Records - Layout of Memory During a Function Call
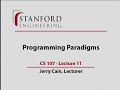
Moving from C Code Generation to C++ Code Generation: Basic Swap Example
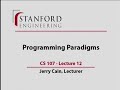
Preprocessing Commands - #Define as a Glorified Find and Replace, Preprocessing Macros
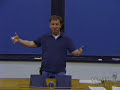
Review of Compilation Process of a Simple Program Into a .O File, Effect of Commenting Out a C Standard Library .H File on the Resulting Translation Unit
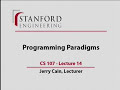
Example in Which Writing Past the End of Array Causes the Return Address of the Function to be Overwritten
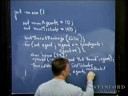
Transitioning from Sequential Programming to Concurrent Programming in the Ticket Sale Example
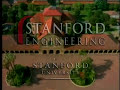
Review of Semaphore Syntax, Semaphoresignal and Semaphorewait
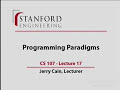
Review of the Dining Philosopher Problem, Modeling Each Philosopher as a Thread
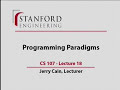
Guest Lecturer, Setup of the Ice Cream Store Problem, with Customer, Cashier, Clerk, and Manager Threads
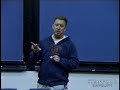
Imperative/Procedural Paradigms (C) and Object-Oriented Paradigm(C++), Introduction to the Functional Paradigm (Scheme)
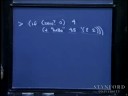
Car-Cdr Recursion Problem that Returns the Sum of Every Element in a List of Integers
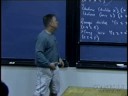
Introduction to the Kawa Development Environment: Evaluation of Expressions, Loading Function Definitions From a .Scm File
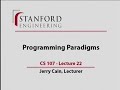
Writing a Recursive Power Set Function in Scheme
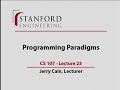
Scheme Memory Model - How Scheme Instructions Synthesize Linked Lists Behind the Scenes and Perform Operations on Them
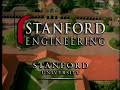
Overarching Features of Python: Scripting Language, Imperative, Object-Oriented, Functional, More Python Overview - Dynamic Typing, Use of Whitespace and Tabs
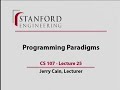
Rewriting RSG to Illustrate all Three Paradigms and Lambdas in Python, How Objects Are Implemented in Python
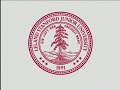
XML Processing and Python - Two Different XML Processing Models, Example XML Fragment
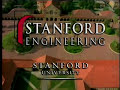
Guest Lecturer: Sasha Rush, Haskell History, Safeguards in Haskell that Avoid Runtime Errors, Expressive Functions in Haskell
関連記事 on Publickey
あわせて読みたい
OpenCourse: Programming Abstractions / Stanford
≪前の記事
OpenCourse: Computer System Engineering / MIT